Bounding box tree¶
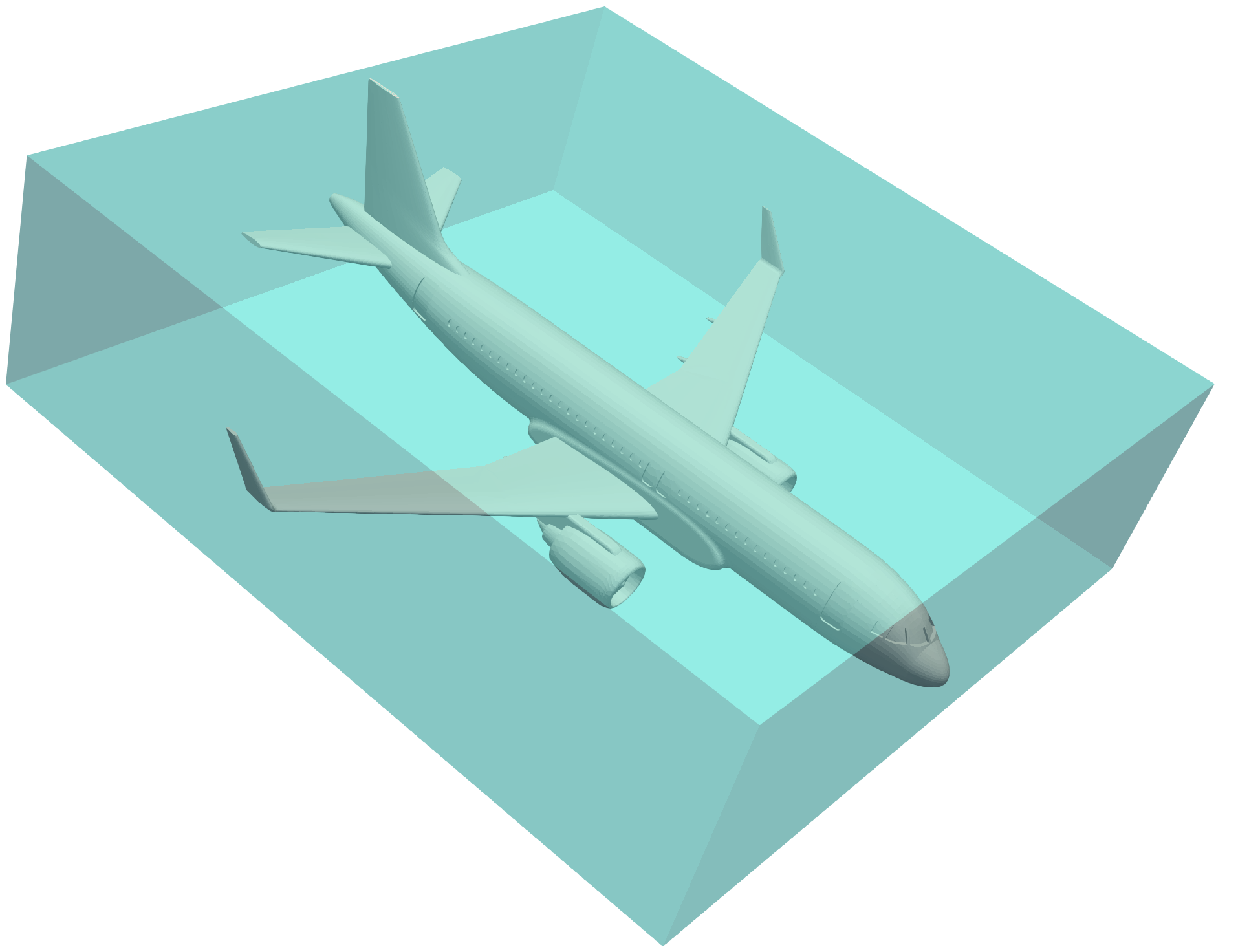
Description¶
Computes an aligned or oriented bounding box tree from the zone’s geometry. A bounding box tree is a hierarchical tree structure where deeper levels confine smaller regions of space. Aligned bounding boxes are boxes that align with the coordinate axis, while oriented bounding boxes do not necessarily align with the coordinate axis.
Parameters¶
- base:
Base
The input base in which compute the bounding boxes
- base:
- oriented_bounding_box: bool, default=
False
If
True
, compute oriented bounding boxes, otherwise compute aligned bounding boxes
- oriented_bounding_box: bool, default=
- tree_level: int, default= 0
The bounding box tree level, 0 is the root level and corresponds to only one bounding box enclosing the whole geometry.
Preconditions¶
The base must have valid coordinate names
Postconditions¶
The output base has as many zones as the input base. The only variables in these zones will be the coordinate. Any other variable is discarded.
If the zone geometry is shared, the output zone will also be in the shared instant and the zone will contain no instants, regardless of the instants in the input zone. If the zone geometry is not shared, then the output zone will contain as many instant as the input instant.
Example¶
import antares
t = antares.Treatment('boundingboxtree')
t['base'] = base
t['oriented_bounding_box'] = True
t['tree_level'] = 2
bbox = t.execute()
or
import antares
bbox = antares.treatment.BoundingBoxTree(
base = base,
oriented_bounding_box = True,
tree_level = 2)
Example¶
Aligned bounding box tree¶
The following example shows how to build an aligned bounding box tree
import antares
import os
output_folder = os.path.join("OUTPUT", "TreatmentBoundingBoxTree")
os.makedirs(output_folder, exist_ok=True)
reader = antares.Reader('hdf_cgns')
reader['filename'] = '../data/A320NEO/a320neo.cgns'
reader['shared'] = False
base = reader.read()
treatment = antares.Treatment('BoundingBoxTree')
treatment['base'] = base
treatment['oriented_bounding_box'] = False
treatment['tree_level'] = 3
aligned_bbox = treatment.execute()
writer = antares.Writer('bin_tp')
writer['base'] = aligned_bbox
writer['filename'] = os.path.join(output_folder, 'aligned_bbox.plt')
writer.dump()
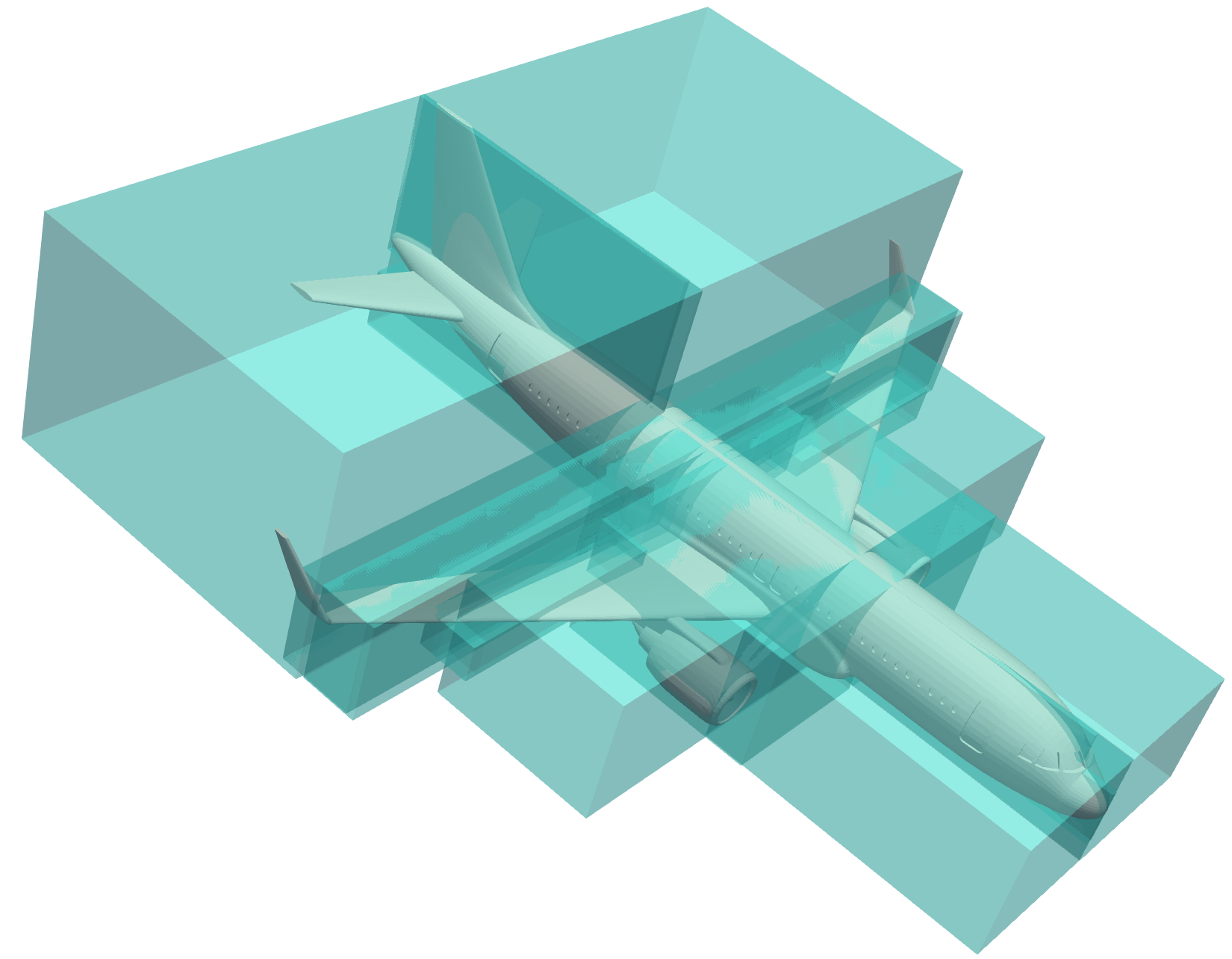
Oriented bounding box tree¶
The following example shows how to build an oriented bounding box tree
import antares
import os
output_folder = os.path.join("OUTPUT", "TreatmentBoundingBoxTree")
os.makedirs(output_folder, exist_ok=True)
reader = antares.Reader('hdf_cgns')
reader['filename'] = '../data/A320NEO/a320neo.cgns'
reader['shared'] = False
base = reader.read()
treatment = antares.Treatment('BoundingBoxTree')
treatment['base'] = base
treatment['oriented_bounding_box'] = True
treatment['tree_level'] = 3
oriented_bbox = treatment.execute()
writer = antares.Writer('bin_tp')
writer['base'] = oriented_bbox
writer['filename'] = os.path.join(output_folder, 'oriented_bbox.plt')
writer.dump()
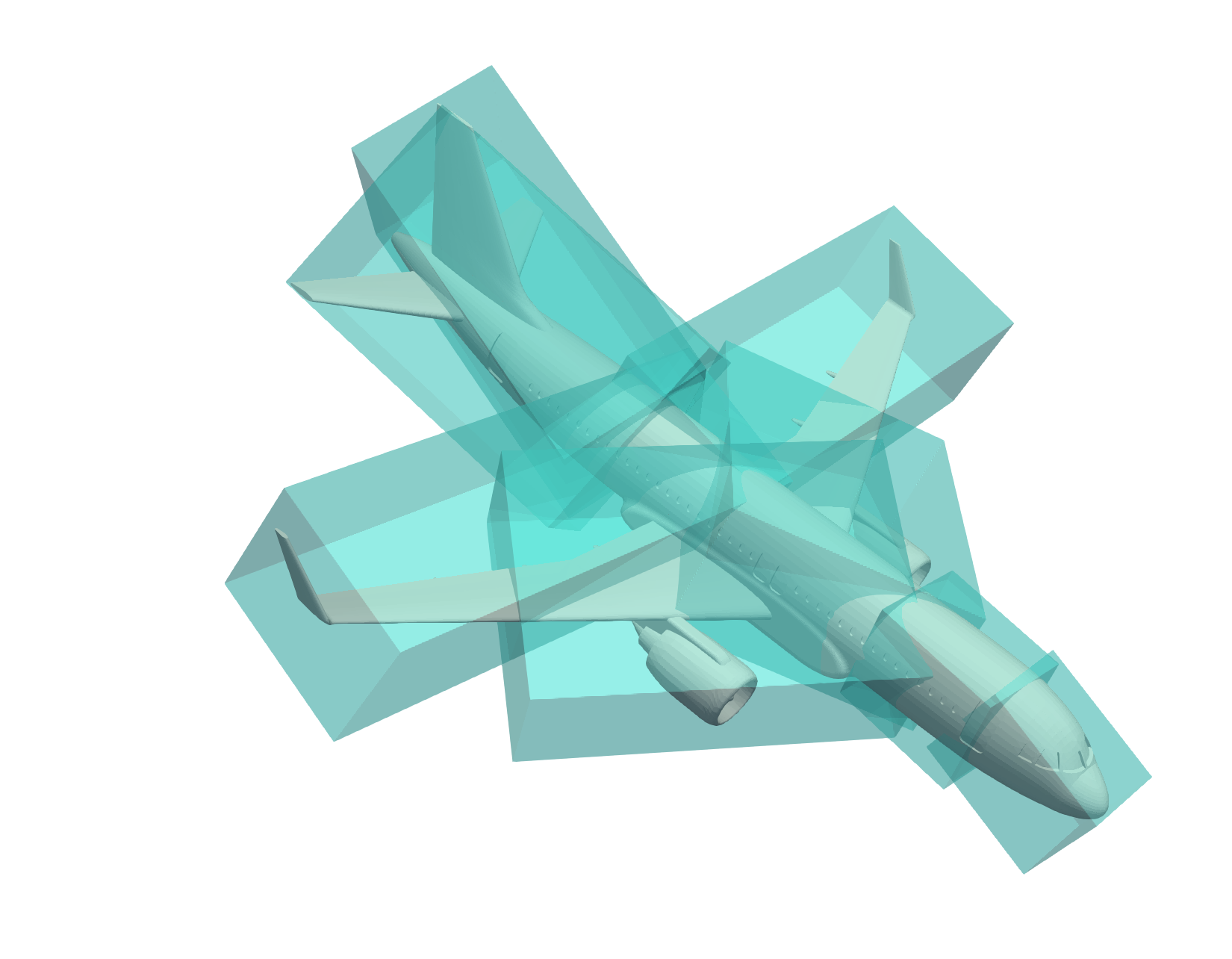