Face-element¶
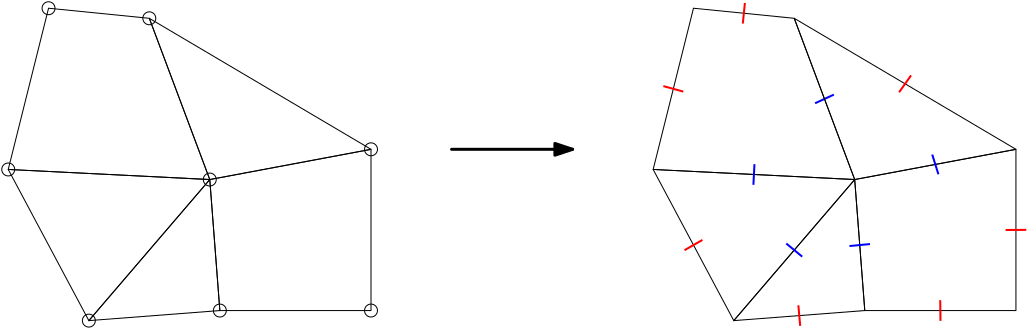
Description¶
Face-element connectivity from the standard element-node connectivity.
In antares, the mesh is represented by the classical element-node connectivity. I.e. for every element type, the nodes of each element for that element type are listed. This treatment converts this representation into a less conventional one: face-element connectivity. For every element face the incident elements are listed. The number of incident elements is either 1 (boundary face) or 2 (internal face).
This treatment supports hybrid meshes.
Parameters¶
- base:
Base
antares Base object
- base:
- zones: List[str], default= None
Names of the zone the face-element connectivity of which will be computed. If not given, all the zones are processed.
- internal: bool, default= False
If True, only the internal faces are considered. The boundary option and this option are mutually exclusive.
- boundary: bool, default= False
If True, only the boundary faces are considered. The internal option and this option are mutually exclusive.
Preconditions¶
Currently, the treatment works only in 2D (i.e. when the faces are edges).
Postconditions¶
A dictionary is returned, the keys of which are the names of the zones and the values are the face-element mappings. These mappings are also given in a dictionary: the keys are the faces given by their nodes, while the values are the elements incident to the faces. Note that the elements are numbered continuously across element types, in the same order as the connectivity dictionary of the zone.
with 'internal' mode True
2---------5---------0 {(0, 7): [0, 1], {(0, 7): [0, 1],
| | 1 / | (7, 1): [0, 3], (7, 1): [0, 3],
| 2 | / | (1, 0): [0], (5, 7): [1, 2]}
| | / 0 | (0, 5): [1],
6---------7---------1 (5, 7): [1, 2],
| | (2, 6): [2],
| 3 | (6, 7): [2],
| | (5, 2): [2],
3---------4 (3, 4): [3],
(4, 1): [3],
(7, 3): [3]}
Main functions¶
- class antares.treatment.TreatmentFaceConnectivities.TreatmentFaceConnectivities¶
Determine face-element connectivity.
- execute() Dict[str, DefaultDict[Tuple[int, int], List[int]]] ¶
Invoke the main algorithm.
- Returns:
element-face connectivity. Incident elements to each unique face are returned. By unique face, we mean a single face for the coinciding faces of neighboring elements. Boundary faces can be recognized by having only one incident element, while internal faces have two.
- Return type:
Dict[str, TFaceElem]
- Raises:
ValueError: If boundary and internal faces are demanded at the same time. Choose one of them.
Example¶
import numpy as np
import antares
from antares.core.CustomDict import CustomDict
import pprint
pp = pprint.PrettyPrinter(depth=4)
"""
Example mesh for faceconnectivities treatment
2---------5---------0
| | 1 / |
| 2 | / |
| | / 0 |
6---------7---------1
| |
| 3 |
| |
3---------4
"""
# Create mesh
base = antares.Base()
base["0000"] = antares.Zone()
base[0].shared.connectivity = CustomDict(
{
'tri': np.array([[0, 7, 1], [7, 0, 5]]),
'qua': np.array([[2, 6, 7, 5], [3, 4, 1, 7]]),
}
)
base[0].shared['x'] = np.array([9, 10, 1, 7, 10, 6, 0, 7])
base[0].shared['y'] = np.array([8, -2, 6, -4, -4, 6, 0, -1])
base[0].shared['z'] = np.array([0, 0, 0, 0, 0, 0, 0, 0])
base[0]['0000'] = antares.Instant()
# Run treatment
treatment = antares.Treatment('faceconnectivities')
treatment['base'] = base
result = treatment.execute()
pp.pprint(result)
"""
Output result:
{'0000': defaultdict(<class 'list'>,
{(0, 5): [1],
(0, 7): [0, 1],
(1, 0): [0],
(2, 6): [2],
(3, 4): [3],
(4, 1): [3],
(5, 2): [2],
(5, 7): [1, 2],
(6, 7): [2],
(7, 1): [0, 3],
(7, 3): [3]})}
"""