Erode field¶
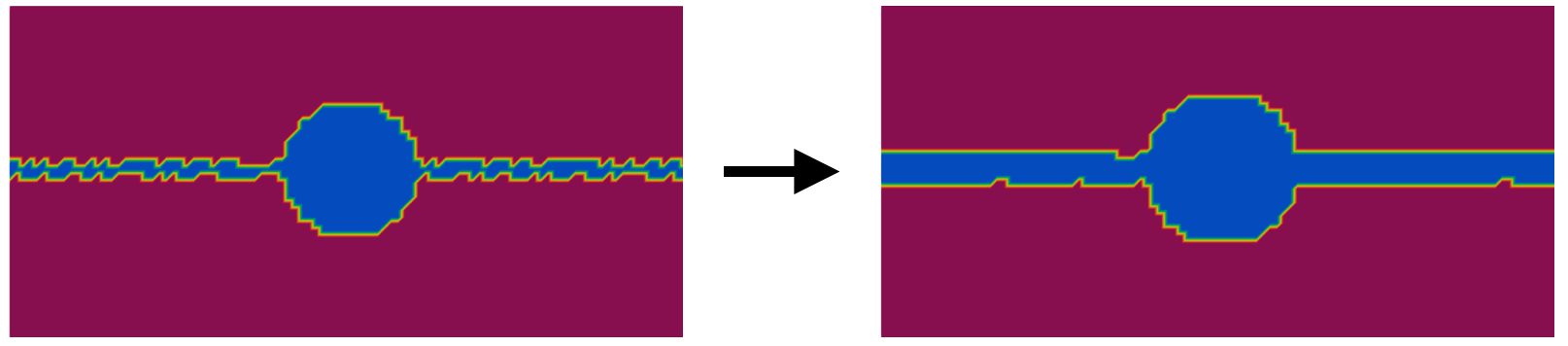
Description¶
The erosion operation propagates the minimum nodal value of a cell to all other nodes of that cell.
Parameters¶
- base:
Base
The input base
- base:
- variables: list(str)
The list of variables to erode
- passes: int, default = 1
The number of times the erode operation is applied to the base
- memory_mode: bool, default =
False
If True, the modifications are done directly on the input base to limit memory usage. If False, a new base is created.
- memory_mode: bool, default =
Preconditions¶
The variables to erode must be located in node.
The base must be unstructured.
Postconditions¶
If memory_mode =
False
, the input base remains unchanged and the output base has the same structure as the input base.If memory_mode =
True
, the input base is modified in-place and the returned base is the same object as the input base.
Usage¶
import antares
treatment = antares.Treatment('erodefield')
treatment['base'] = base
treatment['variables'] = ['var1', 'var2']
treatment['passes'] = 2
eroded_base = treatment.execute()
or
import antares
eroded_base = antares.treatment.ErodeField(
base = base,
variables = ['var1', 'var2'],
passes = 2,)
Example¶
The following example shows the effect of the erode field for 1 pass and 2 passes.
import os
import antares
# Prepare output folder
output_folder = os.path.join("OUTPUT", "TreatmentErode")
os.makedirs(output_folder, exist_ok=True)
# Read example base
base = antares.io.Read(
filename='../data/AMR/amr_example.h5',
format='hdf_antares',)
# Apply erode field treatment for 1 and 2 passes
eroded_base_1_pass = antares.treatment.ErodeField(
base=base,
variables=['metric'],
passes=1)
eroded_base_2_passes = antares.treatment.ErodeField(
base=base,
variables=['metric'],
passes=2)
# Dump bases
antares.io.Dump(
base=eroded_base_1_pass,
filename='eroded_base_1_pass',
folder=output_folder,
format='hdf_antares',)
antares.io.Dump(
base=eroded_base_2_passes,
filename='eroded_base_2_passes',
folder=output_folder,
format='hdf_antares',)
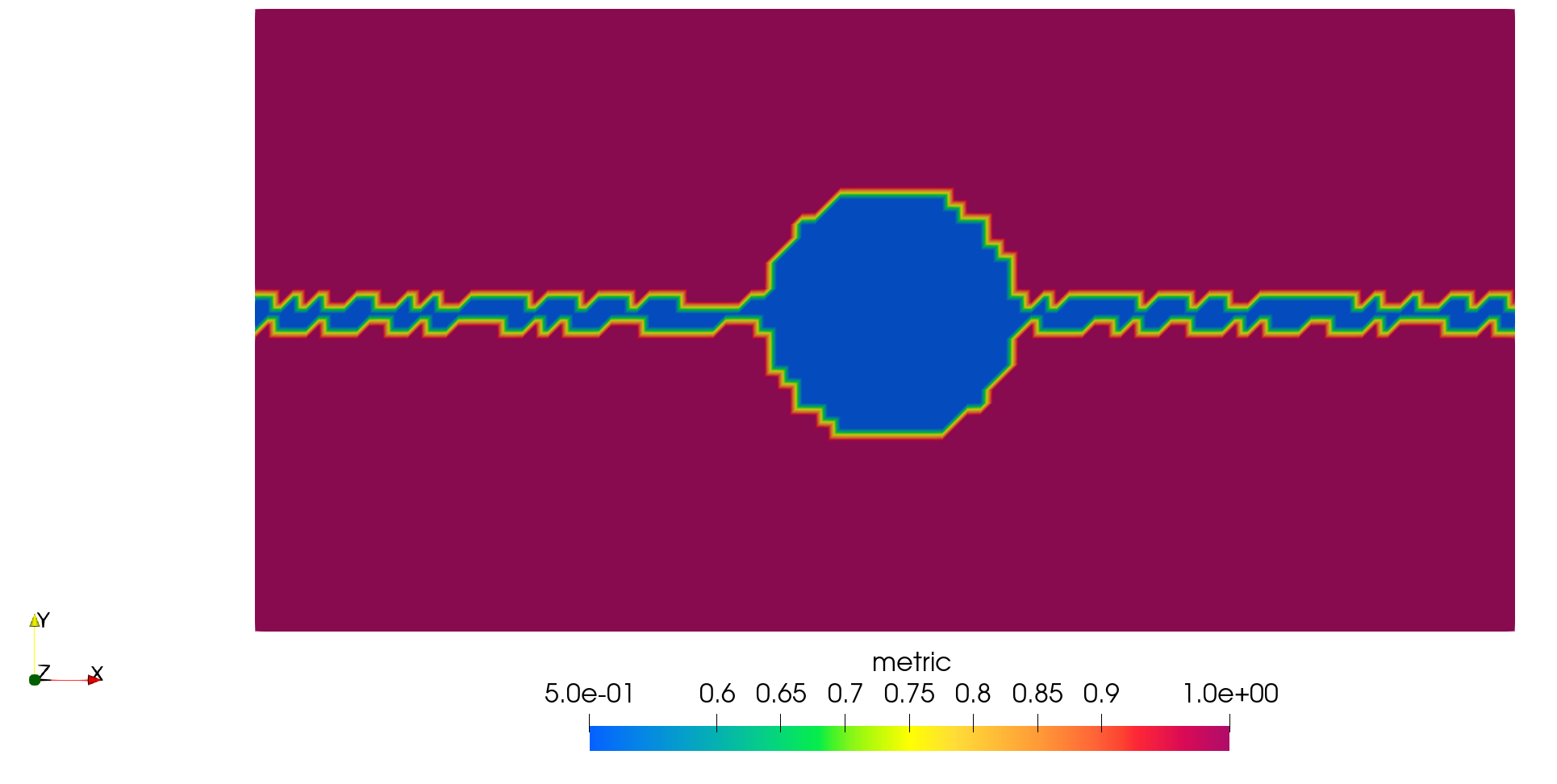
Eroded field after 1 pass
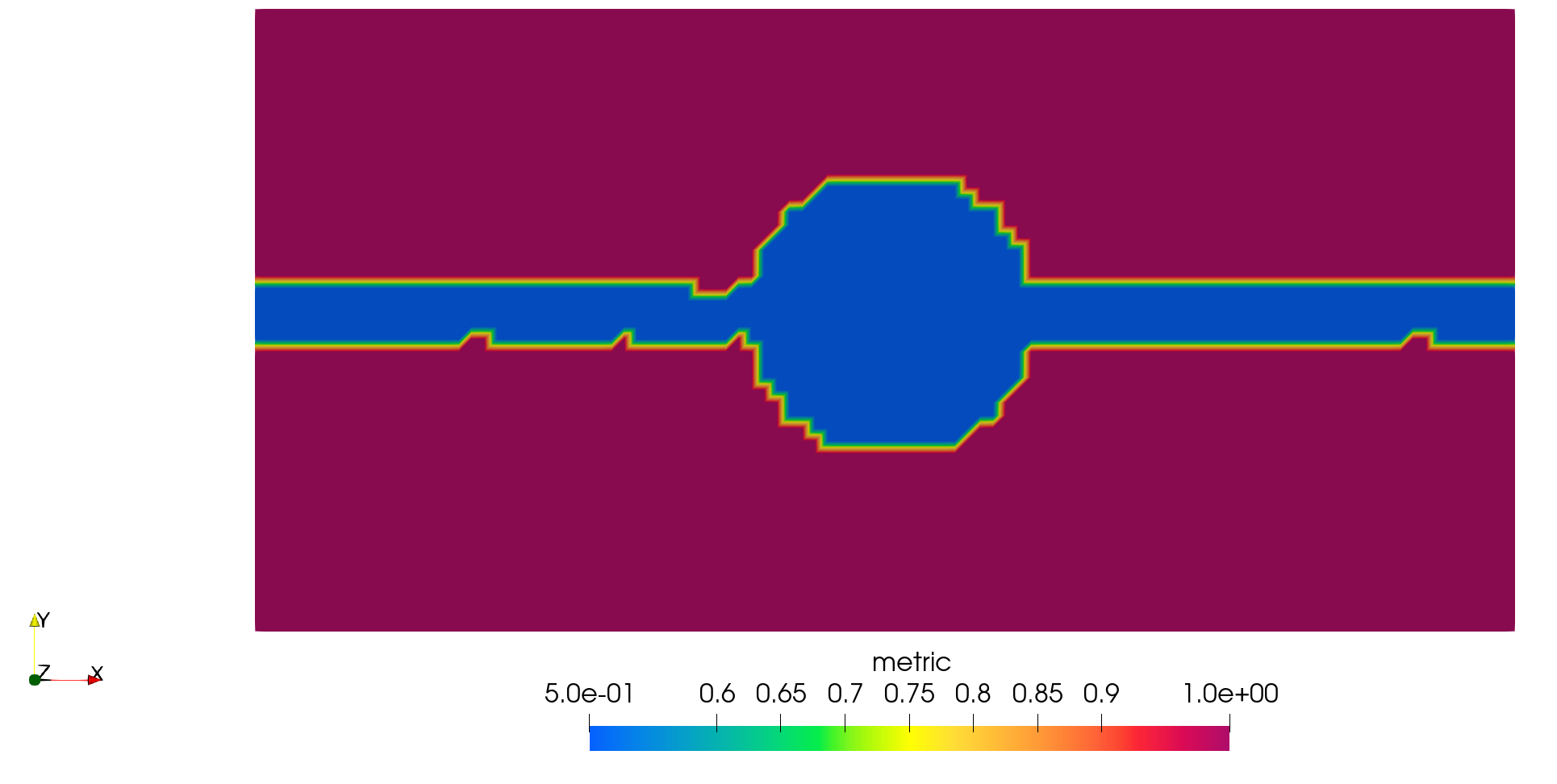
Eroded field after 2 passes
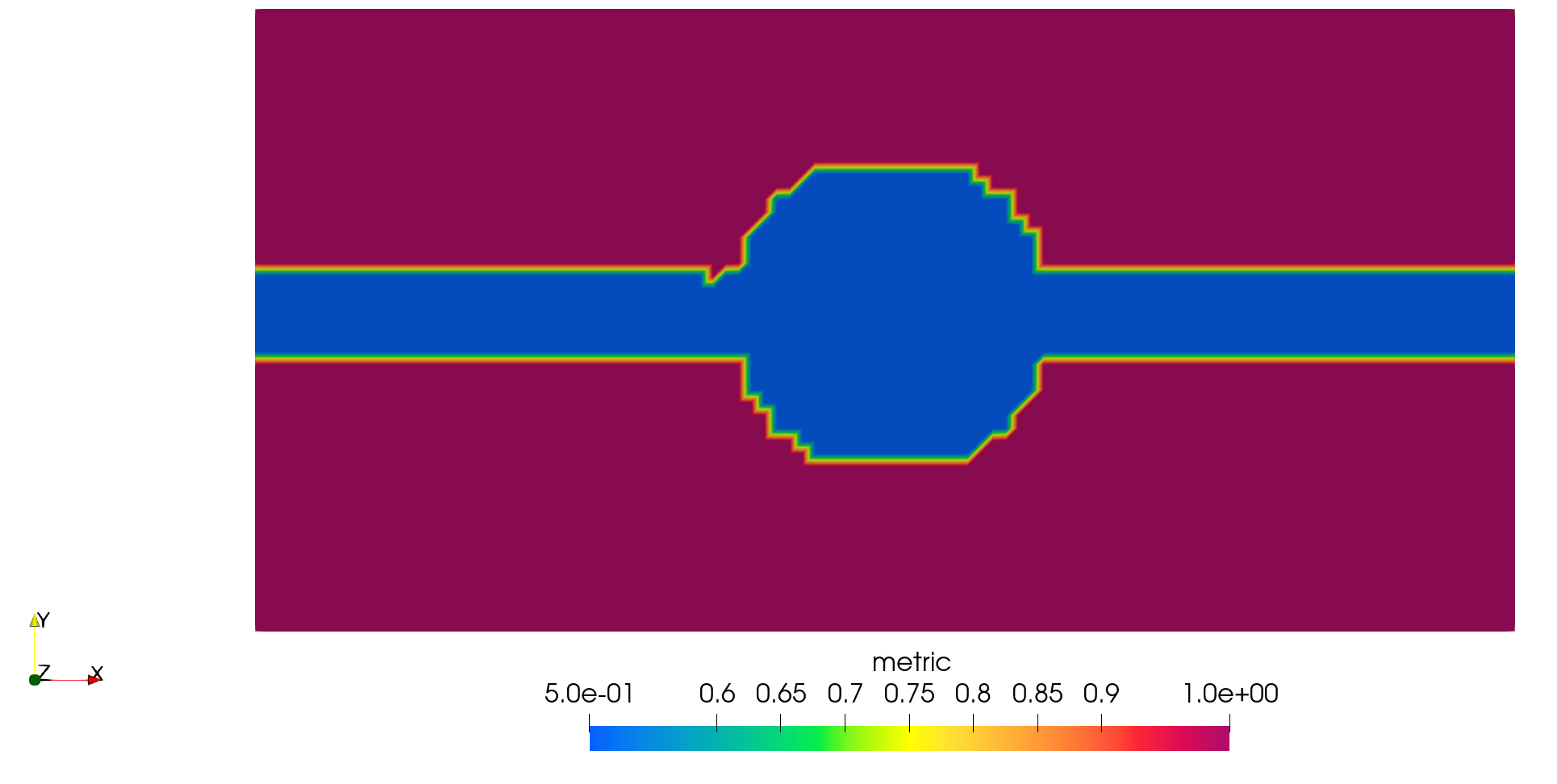